Published
- 4 min read
Difference Between Parallel.ForEach and Parallel.ForEachAsync in C#
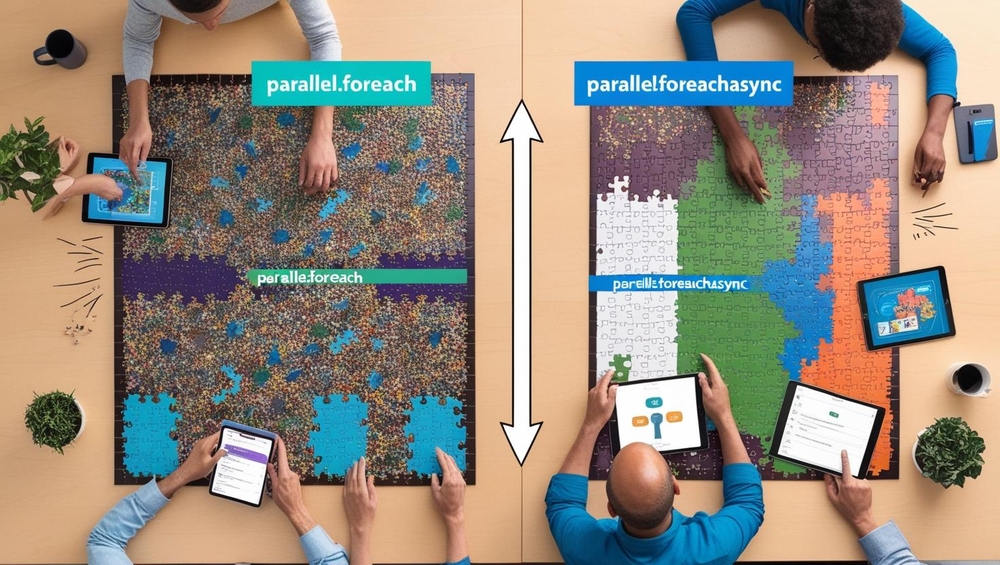
Difference Between Parallel.ForEach and Parallel.ForEachAsync in C#
Understanding the differences between Parallel.ForEach and Parallel.ForEachAsync is crucial for efficient parallel processing in C#.
Introduction
C# provides powerful constructs for parallel and asynchronous programming, allowing developers to write efficient and scalable applications. Two such constructs are Parallel.ForEach
and Parallel.ForEachAsync
. This article explores the differences between these two methods, helping you choose the right one for your use case.
Parallel.ForEach
Parallel.ForEach
is part of the System.Threading.Tasks
namespace and is used for parallel processing of collections. It divides the work among multiple threads, utilizing multiple cores for faster execution.
Key Features
- Synchronous Execution: Executes tasks synchronously across multiple threads.
- Blocking Call: The method blocks the calling thread until all iterations are complete.
- Ideal for CPU-bound Operations: Best suited for operations that are CPU-intensive and do not involve I/O operations.
Example
using System;
using System.Collections.Generic;
using System.Threading.Tasks;
class Program
{
static void Main()
{
var numbers = new List<int> { 1, 2, 3, 4, 5 };
Parallel.ForEach(numbers, number =>
{
Console.WriteLine($"Processing number: {number}");
});
}
}
Real-World Scenario
Consider a scenario where you need to perform complex mathematical calculations on a large dataset. Using Parallel.ForEach
, you can distribute these calculations across multiple threads, significantly reducing the time required to process the entire dataset.
Performance Considerations
When using Parallel.ForEach
, it’s important to consider the overhead of thread management. While it can significantly speed up CPU-bound tasks, the benefits may diminish if the task is not sufficiently parallelizable or if the overhead of managing threads outweighs the performance gains.
Advanced Usage
In more advanced scenarios, Parallel.ForEach
can be combined with other parallel constructs to achieve even greater performance improvements. For example, you can use it in conjunction with PLINQ
(Parallel LINQ) to perform complex data transformations in parallel.
Parallel.ForEachAsync
Parallel.ForEachAsync
is a newer addition to the .NET ecosystem, introduced to handle asynchronous operations more efficiently.
Key Features
- Asynchronous Execution: Executes tasks asynchronously, allowing for non-blocking operations.
- Non-blocking Call: The method does not block the calling thread, making it suitable for I/O-bound operations.
- Ideal for I/O-bound Operations: Best suited for operations that involve I/O, such as network calls or file access.
Example
using System;
using System.Collections.Generic;
using System.Threading.Tasks;
class Program
{
static async Task Main()
{
var numbers = new List<int> { 1, 2, 3, 4, 5 };
await Parallel.ForEachAsync(numbers, async (number, cancellationToken) =>
{
await Task.Delay(1000); // Simulate asynchronous work
Console.WriteLine($"Processing number: {number}");
});
}
}
Real-World Scenario
Imagine you are developing an application that needs to fetch data from multiple web APIs. Using Parallel.ForEachAsync
, you can initiate multiple asynchronous requests simultaneously, improving the overall responsiveness of your application.
Performance Considerations
Parallel.ForEachAsync
is particularly beneficial for I/O-bound tasks where the main bottleneck is waiting for external resources. By not blocking the calling thread, it allows other operations to proceed, improving the overall throughput of the application.
Advanced Usage
For advanced use cases, Parallel.ForEachAsync
can be integrated with other asynchronous patterns, such as async/await
, to create highly responsive applications that can handle a large number of concurrent operations.
Key Differences
- Execution Model:
Parallel.ForEach
is synchronous, whileParallel.ForEachAsync
is asynchronous. - Use Cases: Use
Parallel.ForEach
for CPU-bound tasks andParallel.ForEachAsync
for I/O-bound tasks. - Blocking Behavior:
Parallel.ForEach
blocks the calling thread, whereasParallel.ForEachAsync
does not.
Comparison Table
Feature | Parallel.ForEach | Parallel.ForEachAsync |
---|---|---|
Nature | Synchronous | Asynchronous |
Support for async/await | No | Yes |
Blocking | Blocks the calling thread until complete | Non-blocking; returns a Task |
Best for | CPU-bound tasks | IO-bound or asynchronous tasks |
Cancellation | Supports cancellation tokens | Supports cancellation tokens |
Return Type | void | Task |
Advanced Topics
Task Cancellation
Both Parallel.ForEach
and Parallel.ForEachAsync
support task cancellation through the use of CancellationToken
. Implementing cancellation tokens allows you to gracefully terminate tasks when needed, freeing up resources and improving application responsiveness.
Error Handling
Error handling in parallel and asynchronous operations can be challenging. It’s important to implement robust error handling mechanisms, such as try-catch blocks, to manage exceptions and ensure the stability of your application.
Resource Management
Efficient resource management is crucial when working with parallel and asynchronous operations. Be mindful of memory usage, especially when dealing with large datasets or network operations. Consider using resource pooling techniques to optimize resource allocation and reduce overhead.
Scalability Considerations
When designing applications that use Parallel.ForEach
or Parallel.ForEachAsync
, consider the scalability of your solution. Ensure that your application can handle increased workloads by optimizing resource usage and minimizing bottlenecks.
Best Practices
- Task Cancellation: Always implement cancellation tokens to gracefully handle task cancellations.
- Error Handling: Use try-catch blocks to manage exceptions within parallel loops.
- Resource Management: Be mindful of resource usage, especially when dealing with large datasets or network operations.
- Scalability: Design your application to scale efficiently by optimizing resource usage and minimizing bottlenecks.
Conclusion
Choosing between Parallel.ForEach
and Parallel.ForEachAsync
depends on the nature of your tasks. For CPU-bound operations, Parallel.ForEach
is more suitable, while Parallel.ForEachAsync
is ideal for I/O-bound tasks. Understanding these differences will help you write more efficient and responsive applications in C#.