Published
- 4 min read
Dictionaries in CSharp
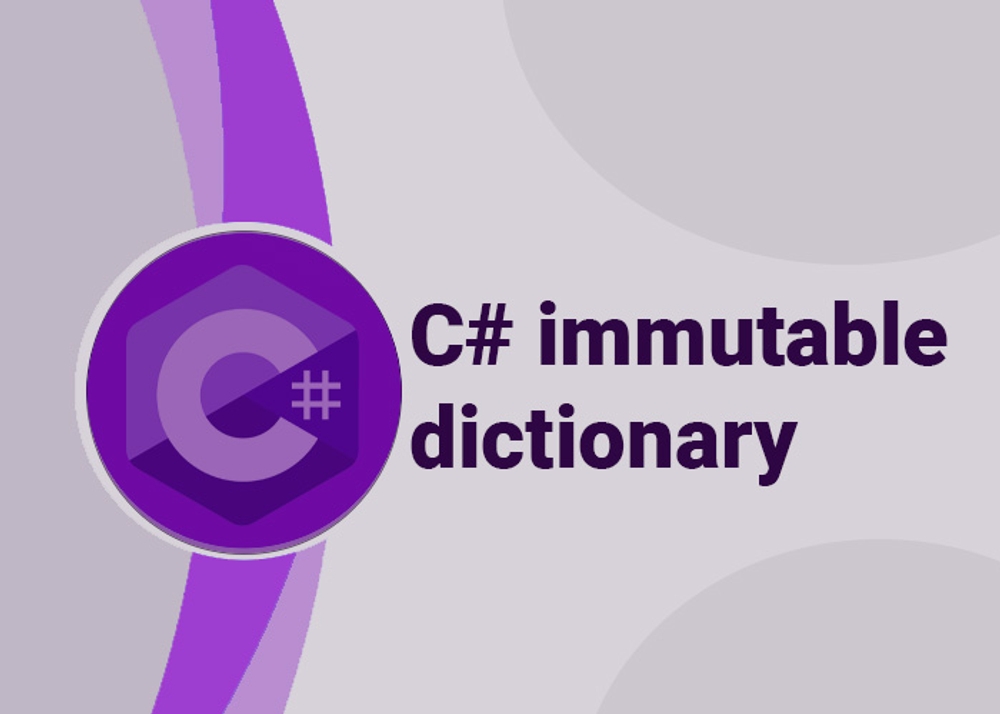
Dictionaries in C#
C# provides a powerful data structure called Dictionary that allows you to store key-value pairs.
Introduction
A dictionary is a collection of key-value pairs. It is a data structure used to store data in the form of key-value pairs. The key-value pair is also referred to as an entry. The key is used to retrieve the data associated with it. The key must be unique and immutable. The value can be changed. The value can be of any type. The key and value can be of the same type or of different types.
Dictionary Characteristics
<TKey, TValue>
stores key-value pairs.- Comes under .Collections.Generic namespace.
- Implements
<TKey, TValue>
interface. - Keys must be unique and cannot be null.
- Values can be null or duplicate.
- Values can be accessed bypassing associated key in the indexer e.g.
myDictionary[key]
. - Elements are stored as
KeyValuePair<TKey, TValue>
objects.
Creating a Dictionary
You can create the <TKey, TValue>
object bypassing the type of keys and values it can store. The following example shows how to create a dictionary and add key-value pairs.
using System;
using System.Collections.Generic;
namespace Dictionary
{
class Program
{
static void Main(string[] args)
{
Dictionary<string, string> capitals = new Dictionary<string, string>();
capitals.Add("England", "London");
capitals.Add("Germany", "Berlin");
capitals.Add("Russia", "Moscow");
capitals.Add("USA", "Washington");
capitals.Add("Ukraine", "Kyiv");
foreach (KeyValuePair<string, string> keyValue in capitals)
{
Console.WriteLine(keyValue.Key + " - " + keyValue.Value);
}
Console.WriteLine("The capital of England is " + capitals["England"]);
Console.WriteLine("The capital of Germany is " + capitals["Germany"]);
Console.WriteLine("The capital of Russia is " + capitals["Russia"]);
Console.WriteLine("The capital of USA is " + capitals["USA"]);
Console.WriteLine("The capital of Ukraine is " + capitals["Ukraine"]);
capitals.Remove("USA");
Console.WriteLine("The capital of USA is " + capitals["USA"]);
}
}
}
Dictionary Class Hierarchy
Dictionary Methods
The <TKey, TValue>
class provides various methods to perform different operations on the dictionary. The following table lists some of the commonly used methods of the <TKey, TValue>
class.
Method | Description |
---|---|
Add(TKey, TValue) | Adds an element with the specified key and value into the dictionary. |
Clear() | Removes all the elements from the dictionary. |
ContainsKey(TKey) | Checks whether the specified key exists in the dictionary. |
ContainsValue(TValue) | Checks whether the specified value exists in the dictionary. |
Remove(TKey) | Removes the element with the specified key from the dictionary. |
TryGetValue(TKey, out TValue) | Gets the value associated with the specified key. |
Count | Gets the number of elements in the dictionary. |
Dictionary Properties
The <TKey, TValue>
class provides various properties to get information about the dictionary. The following table lists some of the commonly used properties of the <TKey, TValue>
class.
Property | Description |
---|---|
Comparer | Gets the <T> that is used to determine equality of keys for the dictionary. |
Keys | Gets a collection containing the keys in the dictionary. |
Values | Gets a collection containing the values in the dictionary. |
Immutable Dictionaries
In addition to the standard Dictionary, C# also provides Immutable Dictionaries. These are dictionaries that cannot be modified after they are created, ensuring thread-safety and preventing unintended modifications.
Creating an Immutable Dictionary
To use Immutable Dictionaries, you need to include the System.Collections.Immutable
namespace. Here’s an example of how to create an Immutable Dictionary:
using System.Collections.Immutable;
var immutableDictionary = ImmutableDictionary<string, int>.Empty
.Add("One", 1)
.Add("Two", 2)
.Add("Three", 3);
Characteristics of Immutable Dictionaries
- Once created, the dictionary cannot be modified.
- Any operation that would modify the dictionary (like Add or Remove) returns a new ImmutableDictionary instance.
- Provides thread-safe read operations without the need for locking.
- Ideal for scenarios where the dictionary content should not change after initialization.
Methods for Immutable Dictionaries
Immutable Dictionaries provide methods similar to regular Dictionaries, but with a key difference: methods that would normally modify the dictionary instead return a new instance.
Method | Description |
---|---|
Add(TKey, TValue) | Returns a new ImmutableDictionary with the specified key and value added. |
Remove(TKey) | Returns a new ImmutableDictionary with the specified key removed. |
SetItem(TKey, TValue) | Returns a new ImmutableDictionary with the specified key-value pair set. |
Performance Considerations
While Immutable Dictionaries provide benefits in terms of safety and predictability, they may have performance implications for large collections or frequent modifications. Each modification operation creates a new instance, which can be memory-intensive for large dictionaries.